Right Start
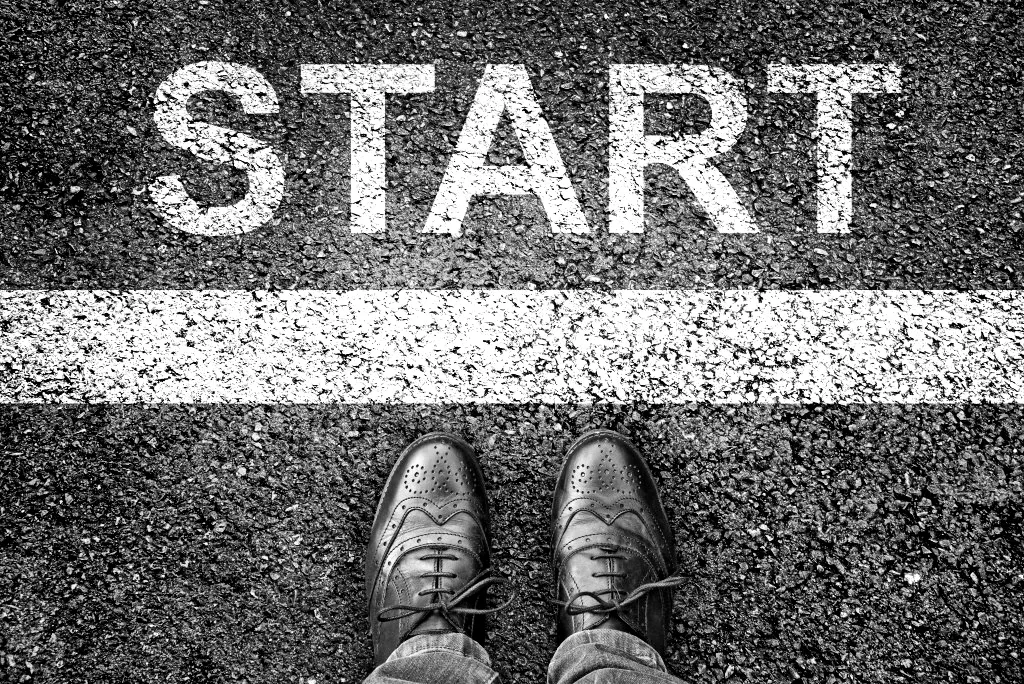
I am really excited to be writing this kick-off post for AppShapes Blog. With so many ideas in the works, so much good stuff to share with you, it is going to be super fun. Many of you who have known me for years remember my previous software company Applanet. You remember how awesome that stuff was...well that was nothing compared to what we are going to see here. I am looking forward to interviewing some of the smartest people in our field, explaining some of the gnarliest tech, and of course...sharing boatloads of wicked lean code. So...let's get after it!
You may be wondering what I have been doing for the past several years, and what kept me from blogging and the conference circuit. In terms writing and speaking it was a combination of my kids needing more of me, my clients needing more of me, and me trying to keep some semblance of self outside of all that. In terms of my clients, I built what became the #1 analytics platform for the derivatives markets. This was one of the largest, most complex, and most fun projects I have ever seen...and that really is saying a lot (remember I have been slinging code for 30+ years). Outside of family and work, I took a detour from rock climbing and yoga and went all in (and I mean all in) on CrossFit. And I want to preach for a moment here: whatever it is for you (e.g., running, walking, hiking) you have got to have a movement practice to balance the intense mental practice of our field. If you don't have something, get it. I really want that for all of you!
Having written that last paragraph I realize there is so much more that needs to be said about what I have been doing "outside" of tech. But that will have to wait for future posts, right now I'm about to burst wanting to write about the real topic of this post: how to start any software project.
Right Path
It is NEVER too late to get a project on the Right Path - but the effort to do so increases in magnitude the longer a project is on the Compromise Path. So at the start of any project, it is in EVERYONE's interest to start off on the Right Path. But what does that mean exactly?
Let's use the common metaphor of a blank canvas to depict the start of our project. The first Right Path thing to do is to extract a list of stories from the project concept. I will write more about lean and agile development in future posts, but for now the important idea is that we want to start off with a very high-level list of things the system does. Resist the urge to be specific, to be low-level - that comes later when stories are separated.
So now we have our canvas filled with stories about what the system does. What now? Well, you know what the system does, at least high level, so get started. But wait!!! This here is where teams get off the Right Path on to the Compromise Path. You're about to write some code - and for now let's set aside whether you write test-driven or behavior-driven or xxx-driven code - you should be thinking now and for the duration of the project about Primal Concerns.
Primal Concerns
Only ten years before I started programming, Dijkstra wrote about separation of concerns - where a "concern" in simplest terms is something tangible that the system does (e.g., presentation, caching, persistence). The basic idea in separation of concerns is that the concerns must perform their function without coupling the specific behavior of any other concern. I will assume that we're all in agreement of the value of separation of concerns. I mean, it's been 40 years...I think we got that one. Now some concerns are special - they are necessarily used throughout the system by other concerns. We call these concerns cross-cutting. And their improper handling is one of the common ways a project ends up on the Compromise Path. But that is also a topic for another post (proper handling of cross-cutting concerns). For now the important point is that we allow for cross-cutting concerns as long as properly handled.
Some cross-cutting concerns are very special - and these I have coined Primal Concerns. They are and should be used by every part of the system. And I call them Primal Concerns because, you guessed it, they need to be there first. The reason is that in order to be professional in our craft, in order for the system to remain on the Right Path, in order for the system to provide the Right Service (another future post), you must use the Primal Concerns from start to "finish". Which leads us back to being ready to write code for the system.
Let's say our software project is an authentication plug-in for ASP.NET applications. Our stories currently include:
When a Principle authenticates, the event is recorded in the Principle Repository.
We see we need a Principle and a Principle Repository. Shall we take a look at the Compromise Path first?
public class PrincipleRepository {
protected virtual IStorage Storage {
get;
}
public PrincipleRepository(IStorage storage) {
Storage = storage;
}
public virtual void Update(IPrinciple principle) {
Storage.Store(principle);
}
}
Now, I am being generous here. There is plenty Right Path stuff already in this version, the most important being an obvious commitment to Single Responsibility Principle (yup, another post). If this looks like your code, I am super happy for you...master craftsman. But there is something amiss here and if our software project continues to look like this then we are at high risk of failure to provide Right Service. Let's look at the Right Path to see what is missing.
public class PrincipleRepository {
private static ILogger Logger {
get;
} = LoggerManager.GetLogger < PrincipleRepository > ();
protected virtual IStorage Storage {
get;
}
public PrincipleRepository(IStorage storage) {
Logger.Info(nameof(PrincipleRepository), $ "Storage: {storage}");
Storage = storage;
}
public virtual void Update(IPrinciple principle) {
Logger.Info(nameof(Update), $ "Principle: {principle}");
Storage.Store(principle);
}
}
As you can see this version includes Logging, but why? What have we gained here? Well, first there is the obvious: when a PrincipleRepository is initialized we log information about the IStorage it was passed. Similarly, when a Principle is being updated we log information about the IPrinciple. Perhaps not so obvious is that we are being covered for what we don't yet know about the system. Logging is a safety net for the unexpected, for change. Logging is a commitment to not predicting where the system will go (you can't really). It is the buck-stops-here of things you can do to keep your software project on the Right Path.
Do you yet know the lifetime management of PrincipleRepository objects? Do you know the impact of one IStorage implementation over another? How will Principle authentication be audited, troubleshot? By committing to Logging as a Primal Concern, you are being as professional as possible. You are saying at all stages of the software that you do NOT know everything about the Right System - but that whatever comes up you will be able to figure it out.
At the start of this post I mentioned one of the things I'd been doing (derivatives analytics), and maybe that seemed orthogonal. Well, it's not. The prime reason that this large, complex software was maintained as the Right System was that there was Logging from the beginning, everywhere. I have years of experience with some of the most high-pressure issues and changes in the system, and without logs I would have been close to useless. The software would have slowly left the Right Path and on to the Compromise Path and then no longer the Right System.
Let's take this from another angle, to really hammer home the point. By now I am seeing software teams embrace unit and integration tests...we ain't there yet but at least most of the software I see has some tests. So it is safe to say that you have experienced the value behind tests. They cover the system. I don't mean that in a statistical sense, I mean that tests are your safety net to unexpected issues and change. With tests in place, we can trust that the system will be predictable and that we will know early the impact of any set of issues and changes. I am telling you that logs are similar to tests: they are prime tools to keeping your system on Right Path and providing Right Service.
Where We Go From Here Is a Choice I Leave to You
The real meat of this post is about Right Path. Of all the ways I have served my clients, colleagues, readers - talking about and committing myself to Right Path has by far been the most beneficial. So just like a software project has a Right Start, the same goes for this AppShapes Blog. How could I NOT start off with writing a little bit about Right Path??
There is so much, much more to the concept of keeping a software project on the Right Path. I think this post went well in that some key concepts were introduced so future posts can refer back to this one.
So where do we go from here? Well, I can tell you where I DON'T want it to go. I don't want this blog to be a simplex conversation. I want this to be about truly providing value, and so where we go from here is a choice I leave to you. Hit me up on social media and let me know. Be sure to like/follow if you want to see more of what we're doing here.
(The post is over...go do something outside.)